“`json
{
“meta_title”: “How to Improve Website Speed for Better SEO”,
“meta_description”: “Learn effective website speed optimization strategies to boost SEO, enhance user experience, and increase search rankings.”,
“article”: {
“title”: “How to Improve Website Speed for Better SEO”,
“introduction”: “Website speed has become a critical factor for search engine rankings and user experience. In today’s fast-paced digital landscape, users demand websites that load in mere seconds, and search engines reward those that prioritize performance. In this comprehensive guide, we explore actionable website speed optimization techniques to improve your site’s speed and elevate its SEO performance.”,
“sections”: [
{
“heading”: “Why Website Speed Matters for SEO”,
“content”: “Website speed directly impacts user satisfaction and search engine rankings. Slow-loading sites lead to higher bounce rates, lower engagement, and fewer conversions. Moreover, Google’s algorithms prioritize websites with fast load times, making speed optimization a cornerstone of SEO success. Beyond rankings, faster websites create better user experiences, increasing trust and loyalty among visitors.”
},
{
“heading”: “How to Measure Your Website’s Speed”,
“subsections”: [
{
“sub_heading”: “Using Google PageSpeed Insights”,
“content”: “Google PageSpeed Insights is a free tool that evaluates your website’s speed on both mobile and desktop devices. It provides detailed reports on performance, identifying areas for improvement. Utilize this tool to pinpoint issues and start optimizing.”
},
{
“sub_heading”: “Leveraging GTmetrix”,
“content”: “GTmetrix offers in-depth website analysis, including page load times, file sizes, and performance scores. It provides actionable recommendations, making it invaluable for website speed optimization efforts.”
}
]
},
{
“heading”: “Top Strategies for Website Speed Optimization”,
“subsections”: [
{
“sub_heading”: “Optimize Images”,
“content”: “Large, unoptimized images are among the most common culprits of slow-loading websites. Resize images to appropriate dimensions, compress them using tools like TinyPNG or ImageOptim, and use modern formats such as WebP to reduce file sizes without sacrificing quality.”
},
{
“sub_heading”: “Minimize HTTP Requests”,
“content”: “Each asset on your website (CSS files, JavaScript, images) requires an HTTP request. Reducing the number of requests by combining files, using inline CSS, or removing unnecessary assets significantly enhances website speed.”
},
{
“sub_heading”: “Enable Browser Caching”,
“content”: “Browser caching allows returning visitors to load your website faster by storing static files locally. Configure caching by modifying your server settings or leveraging plugins like W3 Total Cache for WordPress.”
},
{
“sub_heading”: “Optimize Web Hosting”,
“content”: “Your choice of web hosting provider significantly influences website speed. Opt for premium hosting solutions or consider upgrading to dedicated servers or managed WordPress hosting for superior performance.”
},
{
“sub_heading”: “Implement a Content Delivery Network (CDN)”,
“content”: “A CDN distributes website assets across multiple servers worldwide, ensuring faster delivery to users regardless of their location. Popular CDN providers like Cloudflare or AWS CloudFront are highly effective for website speed optimization.”
},
{
“sub_heading”: “Use Lazy Loading”,
“content”: “Lazy loading ensures that images and videos load only when they appear in the user’s viewport. This strategy drastically reduces initial page load time and improves mobile performance.”
},
{
“sub_heading”: “Reduce Server Response Time”,
“content”: “Slow server response times hinder website performance. Optimize your server settings, invest in high-speed hosting, or use tools like PHP opcode caching to lower server latency.”
}
]
},
{
“heading”: “Technical Considerations for Speed Optimization”,
“subsections”: [
{
“sub_heading”: “Minify CSS, JavaScript, and HTML”,
“content”: “Minification removes unnecessary spaces, line breaks, and comments from code to reduce file sizes. Use tools like Gulp or plugins like Autoptimize for WordPress to streamline this process.”
},
{
“sub_heading”: “Implement AMP (Accelerated Mobile Pages)”,
“content”: “AMP ensures lightning-fast load times on mobile devices by delivering simplified versions of your web pages. This technology particularly benefits publishers and ecommerce websites seeking better performance on mobile.”
}
]
},
{
“heading”: “Common Website Speed Optimization Mistakes to Avoid”,
“content”: [
“Overloading your website with plugins that increase bloat.”,
“Ignoring mobile-first design principles.”,
“Failing to regularly audit website speed and performance.”
]
},
{
“heading”: “Monitoring Website Speed Over Time”,
“content”: “Consistent monitoring is crucial to maintaining optimal website speed. Schedule routine audits using tools like Pingdom or Lighthouse to identify and rectify emerging issues. Performance trends offer invaluable insights into how your website evolves over time.”
},
{
“heading”: “Conclusion”,
“content”: “Website speed is not just a technical requirement—it’s a gateway to better SEO and a stronger online presence. By implementing these website speed optimization strategies, you’ll ensure a faster, more enjoyable experience for your visitors while boosting your search engine rankings. Stay proactive in monitoring and improving your site’s performance to maintain a competitive edge in the digital ecosystem.”
}
]
}
}
“`
How to Improve Website Speed for Better SEO
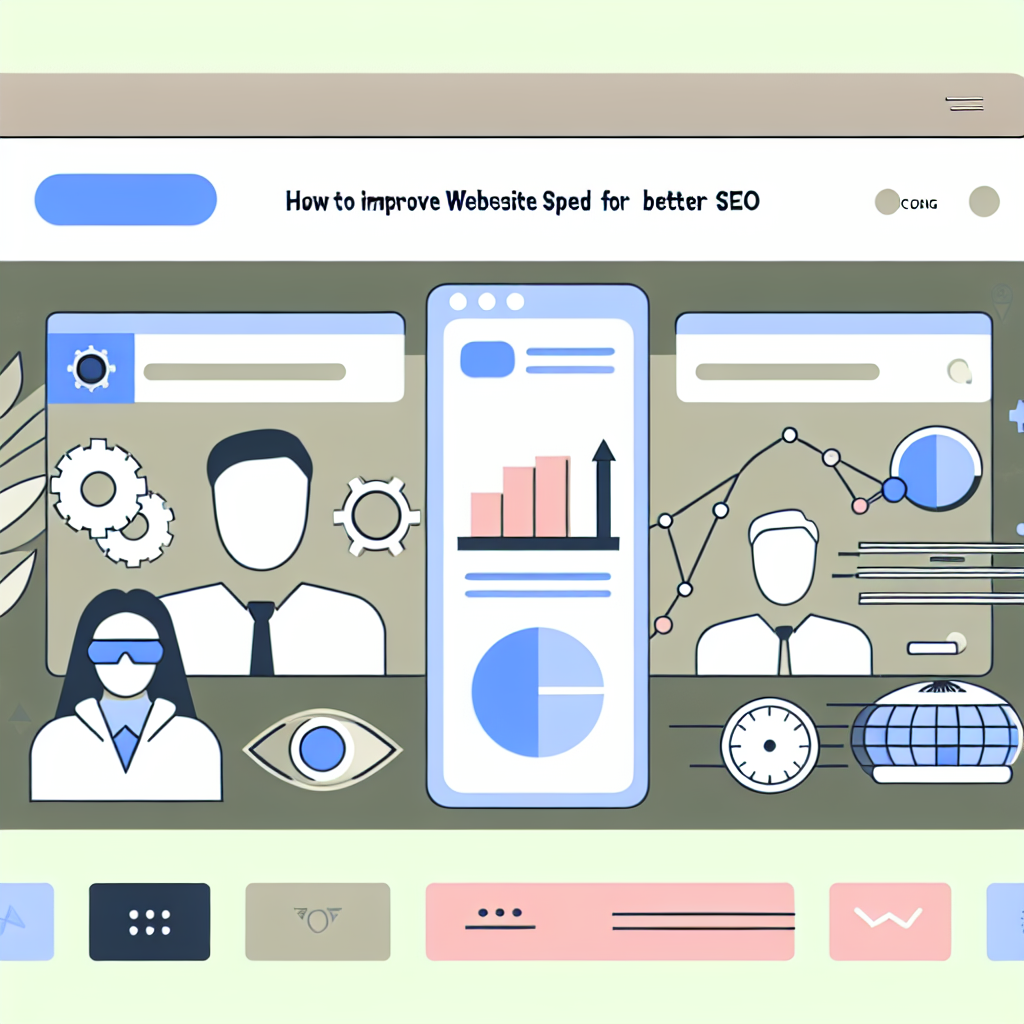